Lesson 2: Controls for input
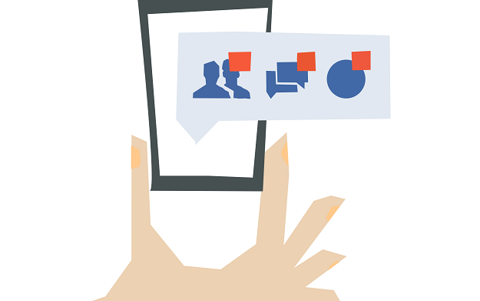
About this lesson
This is the second in a series of lessons to incorporate graphical user interfaces (GUIs) into your general-purpose programming. The series follows on from the Visual To Text Coding lesson series.
Year band: 7-8
Curriculum Links AssessmentCurriculum Links
Links with Digital Technologies Curriculum Area
Strand | Year | Content Description |
---|---|---|
Processes and Production Skills | 7-8 |
Design the user experience of a digital system (AC9TDI8P07) Generate, modify, communicate and evaluate alternative designs (AC9TDI8P08) Design algorithms involving nested control structures and represent them using flowcharts and pseudocode (AC9TDI8P05) Trace algorithms to predict output for a given input and to identify errors (AC9TDI8P06) Implement, modify and debug programs involving control structures and functions in a general-purpose programming language (AC9TDI8P09) |
Assessment
Students undertake a self-reflection of the programming task. The teacher can use the completed self-assessments to assist in summative assessment.
In assessing code in languages like Python or JavaScript, consider a rubric that includes important skills for general-purpose programming.
Learning hook
As part of a graphical user interface (GUI), input controls are the different elements available to your user for providing information and issuing commands, such as buttons, text fields or checkboxes.
Students should start by completing the worksheet ‘Choosing the best input control’. Download the worksheet (Word/PDF).
Now, discuss:
- When do you think a dropdown control is more useful than a text field control, and when is the reverse true?
- When is a spinner/updown control more useful than a text field control?
- Some of these controls have been around for decades. What other more recent GUI elements have you seen on websites or apps?
Note: The activity above touches on the first of four principles for good user interface design (distilled from this article hosted by Adobe). For users to feel in control, a GUI should be:
- easy to navigate (with visual cues that make actions predictable)
- acknowledging (giving feedback and status)
- accommodating of different skill levels
- forgiving (e.g. include an ‘undo’ function)
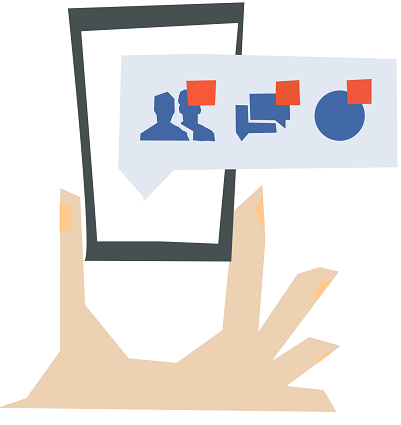
Image: mohamed Hassan/pixabay
Learning map and outcomes
In this lesson, students will:
- access an online programming environment for JavaScript alongside HTML and CSS
- upgrade three text applications to GUI applications with input controls
- take on a fresh coding challenge to explore and incorporate an input control of their choice.
Learning input
This video introduces the first application for this lesson.
Test out the original JavaScript program without a GUI, so you can see our starting point.
Learning construction
Step 1: Setup
In this course, different environments will be selected based on their suitability for each demonstrated project.
For more on the set-up and environments used, see Lesson 1.
Step 2: Upgrading the wi-fi checker to GUI interface
The video below demonstrates changing the wi-fi checker from a text-only program to one with a GUI.
Let's start with some terms. Try it yourself before checking the solution code.
Solution Code
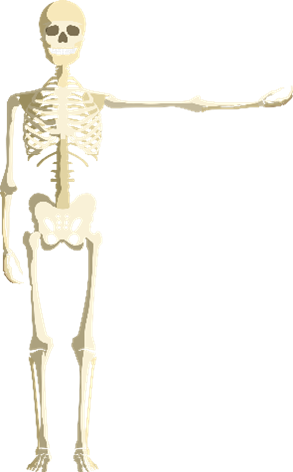
HTML is one of the earliest languages of the Internet. It defines the structure of a webpage, the static content that will appear on the page when it first loads. It also allows for links. You can make a webpage purely in HTML, but it's hard to make it look good!
View this article which answers a common question, Does web development meet the requirements of the Australian Curriculum: Digital Technologies?
You might think of HTML as the skeleton of a webpage.
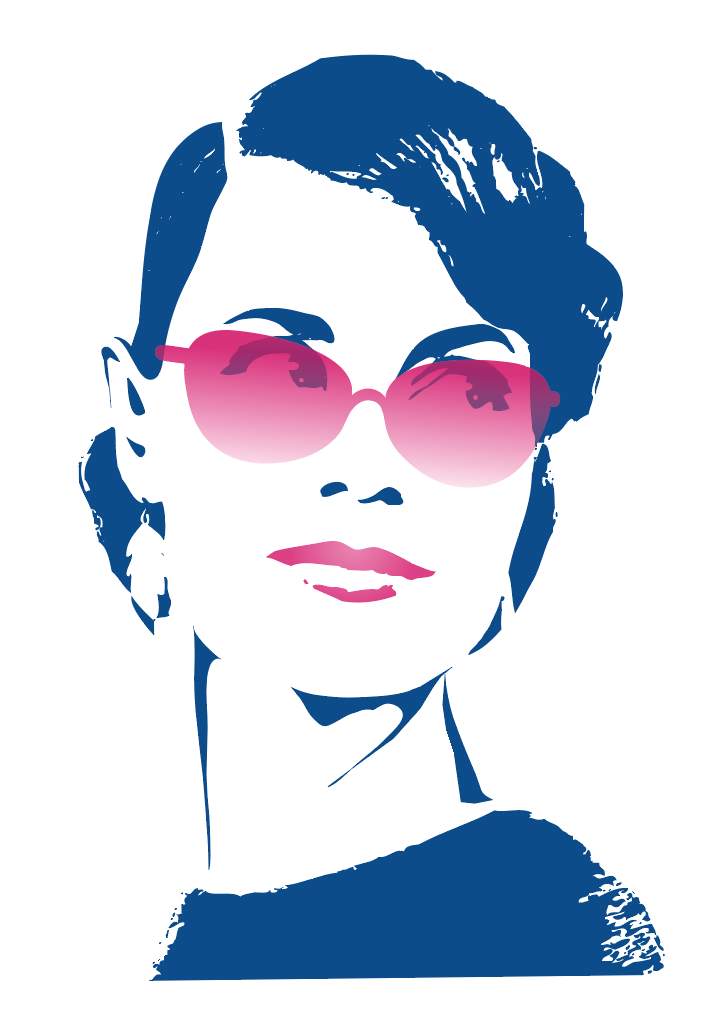
CSS is a script that defines the style of a webpage, allowing for webpages to have interesting and modern look-and-feel: colours, fonts, curved borders, etc.
You might think of CSS as the skin and clothes of a webpage.
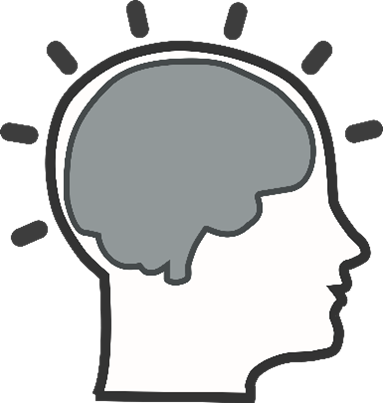
JavaScript defines the functionality of a webpage, allowing interactivity beyond just links. It is the only true general purpose programming language of the three. With JavaScript, webpages can have all the functionality of applications; with loops, decisions, functions and data structures.
You might think of JavaScript as the brain, muscles and nerves of a webpage.
Step 3: Adding a spinner/updown control
The video below further improves the wi-fi checker by adding a spinner/updown control, which gives an instant response.
The video below further improves the wi-fi checker by adding a spinner/updown control, which gives an instant response.
Try implementing this improvement before checking the solution code.
Step 4: Second application – temperature converter
Solution Code
The video below demonstrates upgrading a temperature converter to a GUI.
Try coding this GUI version yourself before checking the solution code.
Step 5: Third application – password generator
Solution Code
The video below demonstrates upgrading a password generator application to a GUI.
Try coding this GUI version yourself before checking the solution code.
Challenge
These challenges use the skills covered so far. By writing or modifying their own programs, students have an opportunity to demonstrate Application and Creation.
The links opposite are a simple text-only application for converting currency. Test it out before continuing.
Your task is to upgrade this application to have a GUI using HTML, JavaScript and CSS. You can choose the best way to receive the user input and to present the result. (Note: your application does not have to use live currency conversion rates.)
- Start by sketching a rough GUI mock-up to show what the user will see and interact with on the webpage. Include text labels and fields, buttons and other input controls.
- Develop the webpage from the text-only starting point, using your preferred environment for HTML, CSS and JavaScript.
Sample solution
Solution Code
This video gives an overview of one sample solution.
This video demonstrates building the same solution.
Could you improve it further by removing the need for pop-up prompts?
Challenge 2
Mock-ups or wireframes are used during user interface design to visually plan or sketch the basic look and functionality.
Sometimes multiple ‘frames’ will be drawn, to show how the interface changes when the user navigates and makes choices within it.
Image: Firmbee/pixabay
Importantly, mock-ups can be annotated with notes and arrows to explain details about the look and feel (e.g. style, fonts, colours) or to explain functionality (e.g. this option appears when this button is pressed).
Tools for wireframing range from free and simple to highly complex paid tools intended for user experience (UX) professionals.
Some free options for school students:
- Draw on paper. Use one colour for the interface elements and another colour for annotations. Sometimes grid paper can help.
- Free online tool diagrams.net (select + more shapes to add GUI mock-up elements).
- Presentation software (such as Microsoft PowerPoint or Google Slides) tends to allow more freedom of element placement than word-processing software.
This challenge is to create a GUI to allow you to experiment with an input control you haven't tried yet. For example, if you are trying radio buttons/option buttons, you might create a simple application that constructs a movie idea as a sentence based on your selections (e.g. gender of main character, setting, genre).
- Choose an input control to try. You might want to consider one of the controls from the worksheet at the start of this lesson.
- Next, consider what sort of simple application could use that control, and prepare a simple mock-up of the full interface, including title, any images, the input controls and output area.
-
Develop the webpage from scratch, using your preferred environment for HTML, CSS and JavaScript. Or you can ‘fork’ and modify one of the basic starting points at right.
HINT: As in the lesson examples, you can use the HTML <input> tag to make your input control(s). The oninput attribute activates your JavaScript function, which will then get the current value from the input control and use it. Alternatively, you can have a <button> tag to make a button with an onclick attribute pointing to your JavaScript function.
This W3Schools webpage lists the many type attributes that allow different input controls to be made with the <input> tag.
Solution Code
Resources
- Online environments for creating webpages with HTML, CSS and JavaScript:
- JSFiddle – simple interface that hides linking HTML code, also used in Visual To Text Coding lesson series
- repl.it – shows complete HTML to reflect offline approach, and allows uploading of images and other files for use in webpages
- Introductory courses
- HTML tutorial – An introduction to HTML
- CSS tutorial – An introduction to CSS
- Visual To Text Coding lesson series – The predecessor to this learning sequence introduces JavaScript as well as Python.
- JavaScript CheatSheet (Tip: Press the little blue tabs to move Variables, Basics, Strings and Data Types to the top.)