Visual to text coding course
This lesson sequence provides a bridge between visual coding (e.g Scratch) and General Purpose Programming languages (e.g Python or JavaScript).
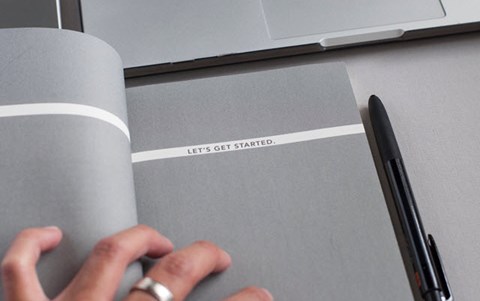
About this course
This lesson sequence provides a bridge between visual coding (eg. Scratch) and General Purpose Programming languages (eg. Python or JavaScript). This resource is most suitable if you have never done General Purpose Programming and/or you benefit from slow-paced, step-by-step video tutorials.
Year band: 5-6, 7-8
Setting Up
30 minutes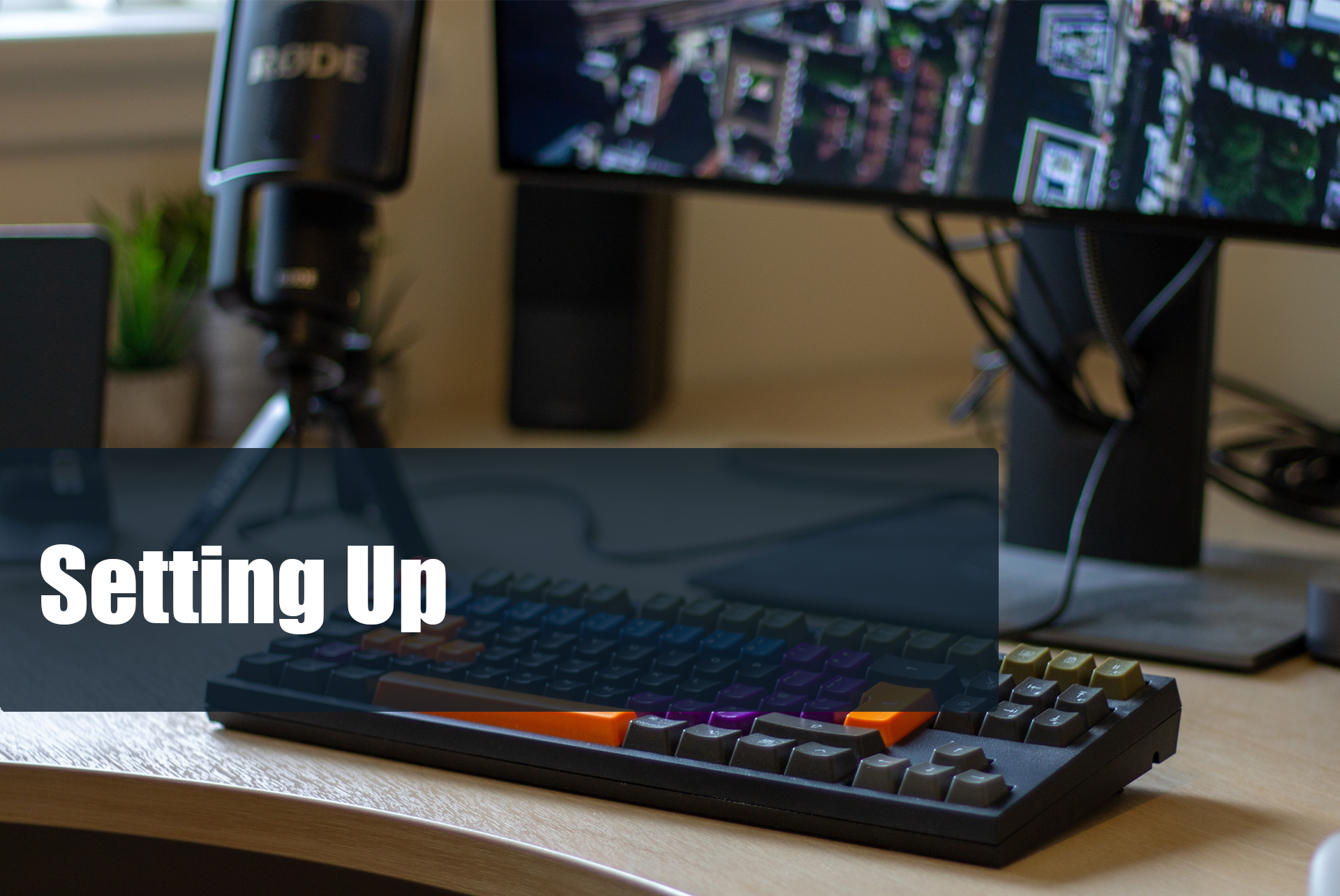
Use these two videos to:
- set up your programming environment.
- go through some of the common errors that people fall victim to!
Videos in this lesson
Setting Up
Gotchas
The Basics
30 MINUTES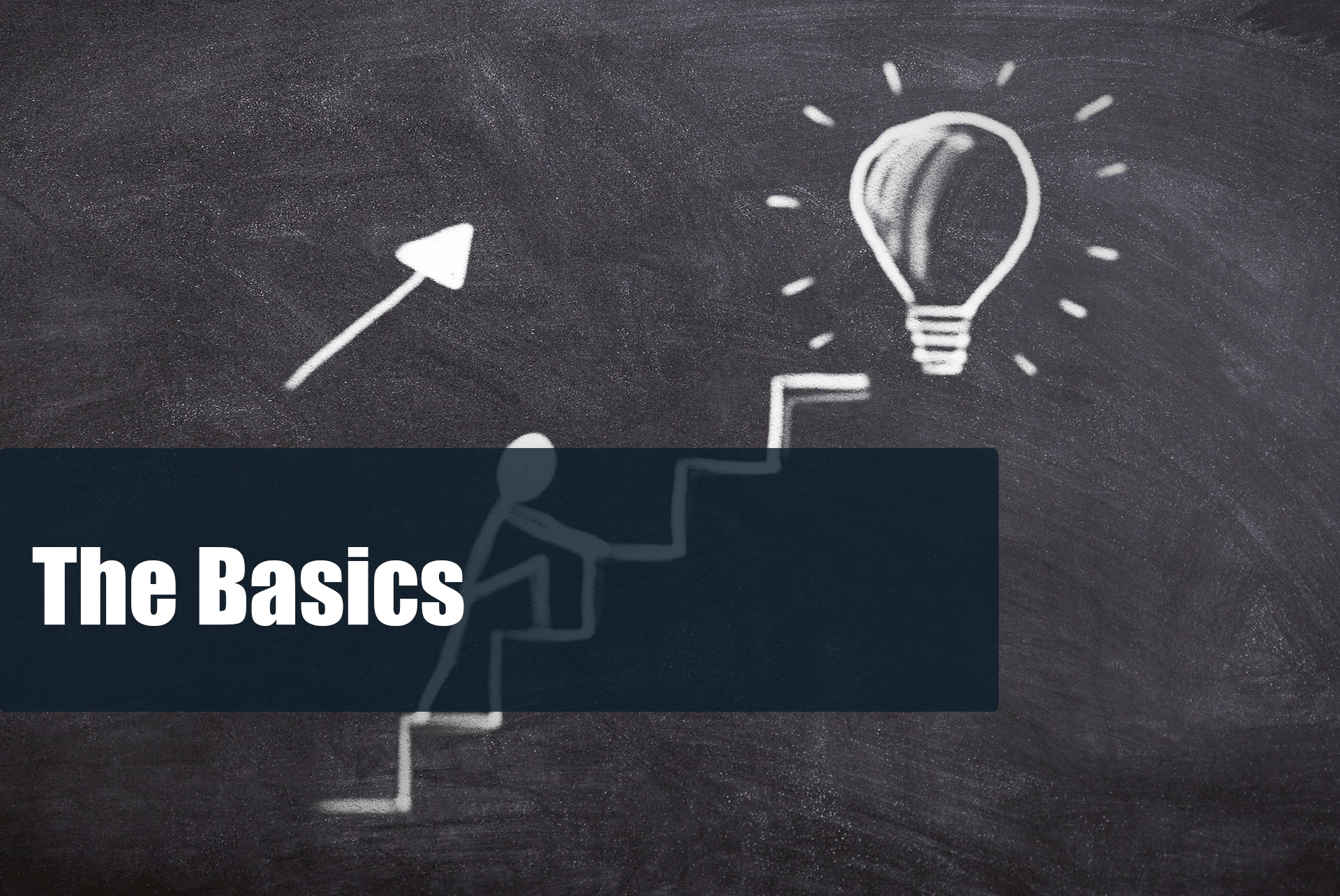
Use these three videos to:
- learn how to store, concatenate and output data.
- build a simple application to consolidate knowledge of these skills
Videos in this lesson
About concatenation
Variables
Lesson 1: Temperature converter
TWO/THREE 45-MINUTE PERIODS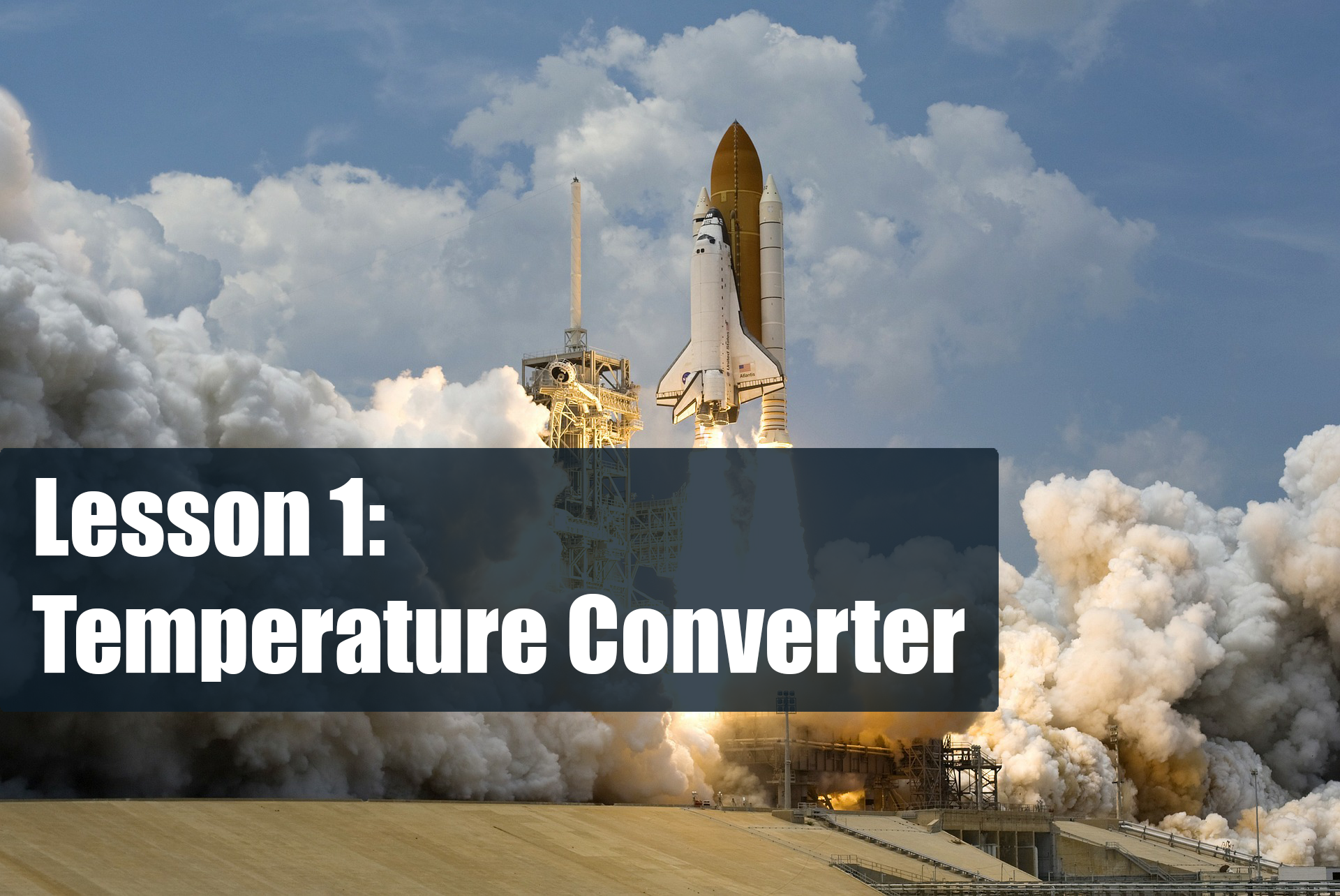
- Use pseudocode for code design.
- Introduce variables.
- Design and code a program to convert temperature units (°C to °F).
- OPTIONAL: Challenge yourself to build other converters, including currency.
Videos in this lesson
Celsius converter
Currency converter
Bitcoin tracker
How much Netflix?
Lesson 2: Calculator
TWO/THREE 45-MINUTE PERIODS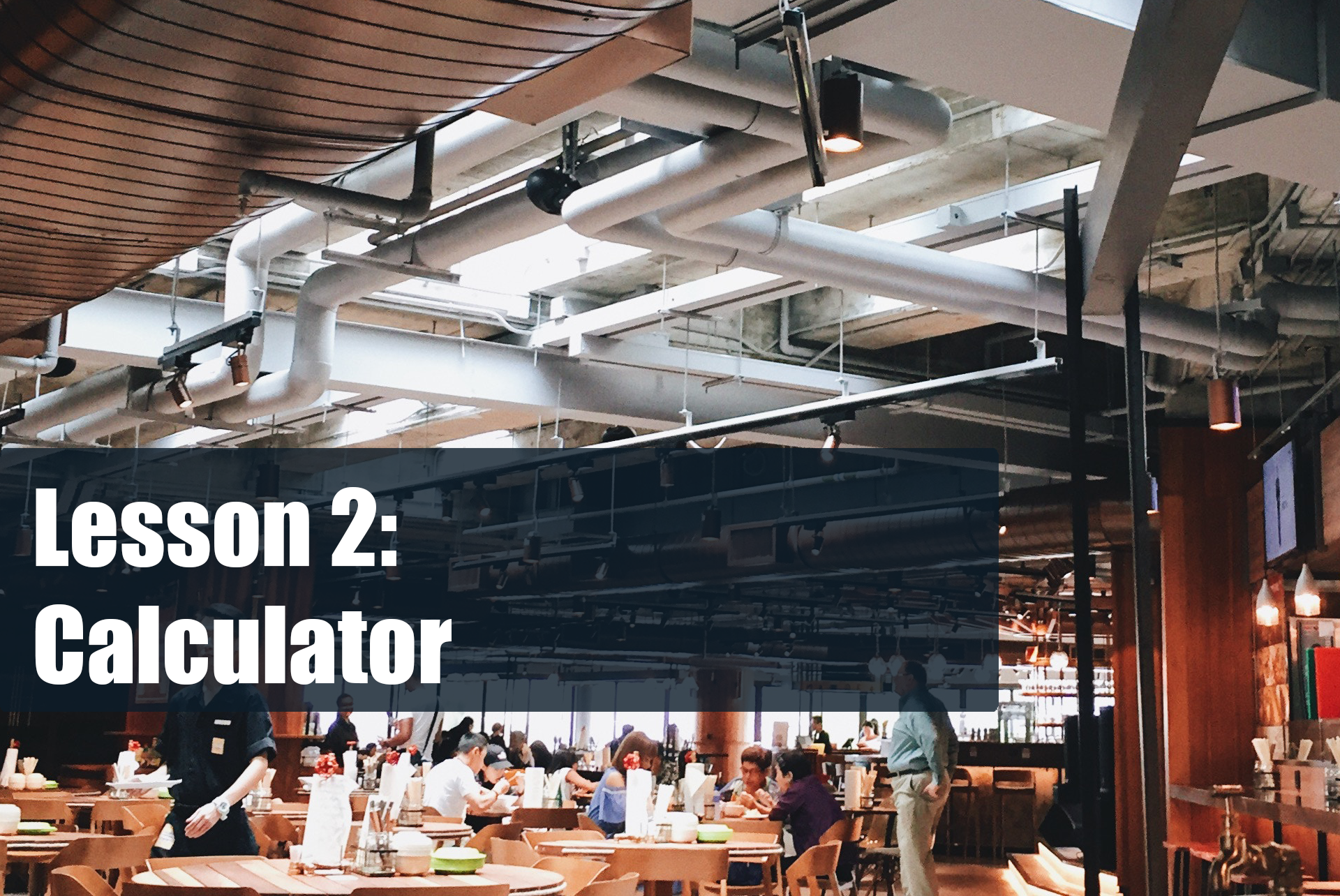
- Introduce branching (decisions).
- Identify data types.
- Design and code a text-based calculator.
- OPTIONAL: Challenge yourself with an ice cream vending interface and a Maths quiz.
Videos in this lesson
Control flow- branching
Calculator
Lesson 3: Heads or Tails
TWO/THREE 45-MINUTE PERIODS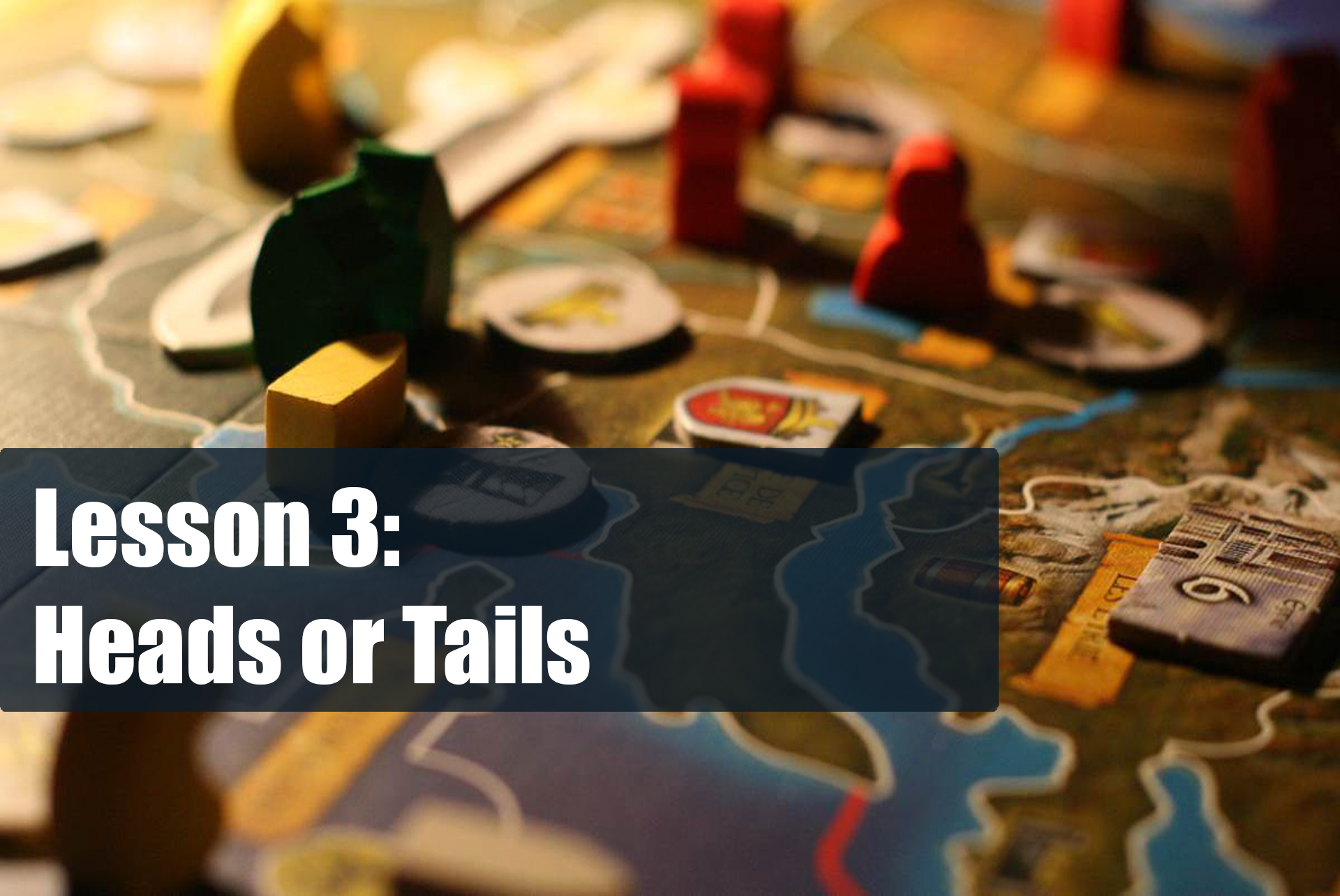
- Introduce random numbers.
- Design and code a heads-or-tails game.
- OPTIONAL: Challenge yourself with a dice roll simulator.
Videos in this lesson
Heads or tails
Dice roll
Lesson 4: Scissors, Paper, Rock
TWO/THREE 45-MINUTE PERIODS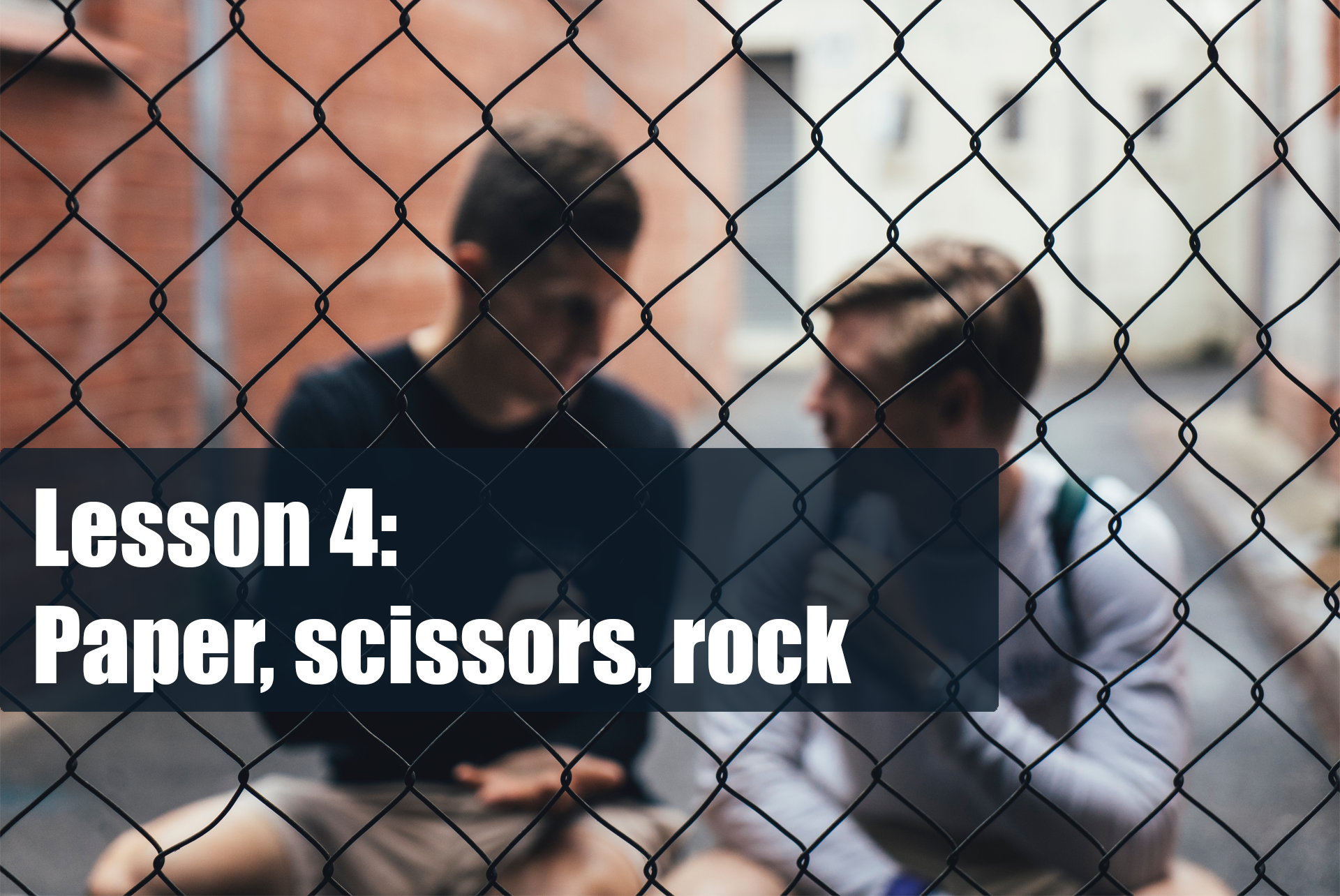
- Introduce combining of logical operators and and or.
- Design and code a game with complex win conditions.
- OPTIONAL: Have a go at designing your own Rock-Paper-Scissors-Lizard-Spock program.
Videos in this lesson
Videos in this lesson: Scissors, Paper, Rock game (overview)
Videos in this lesson: Scissors, Paper, Rock game (coding)
Lesson 5: Password generator
TWO/THREE 45-MINUTE PERIODS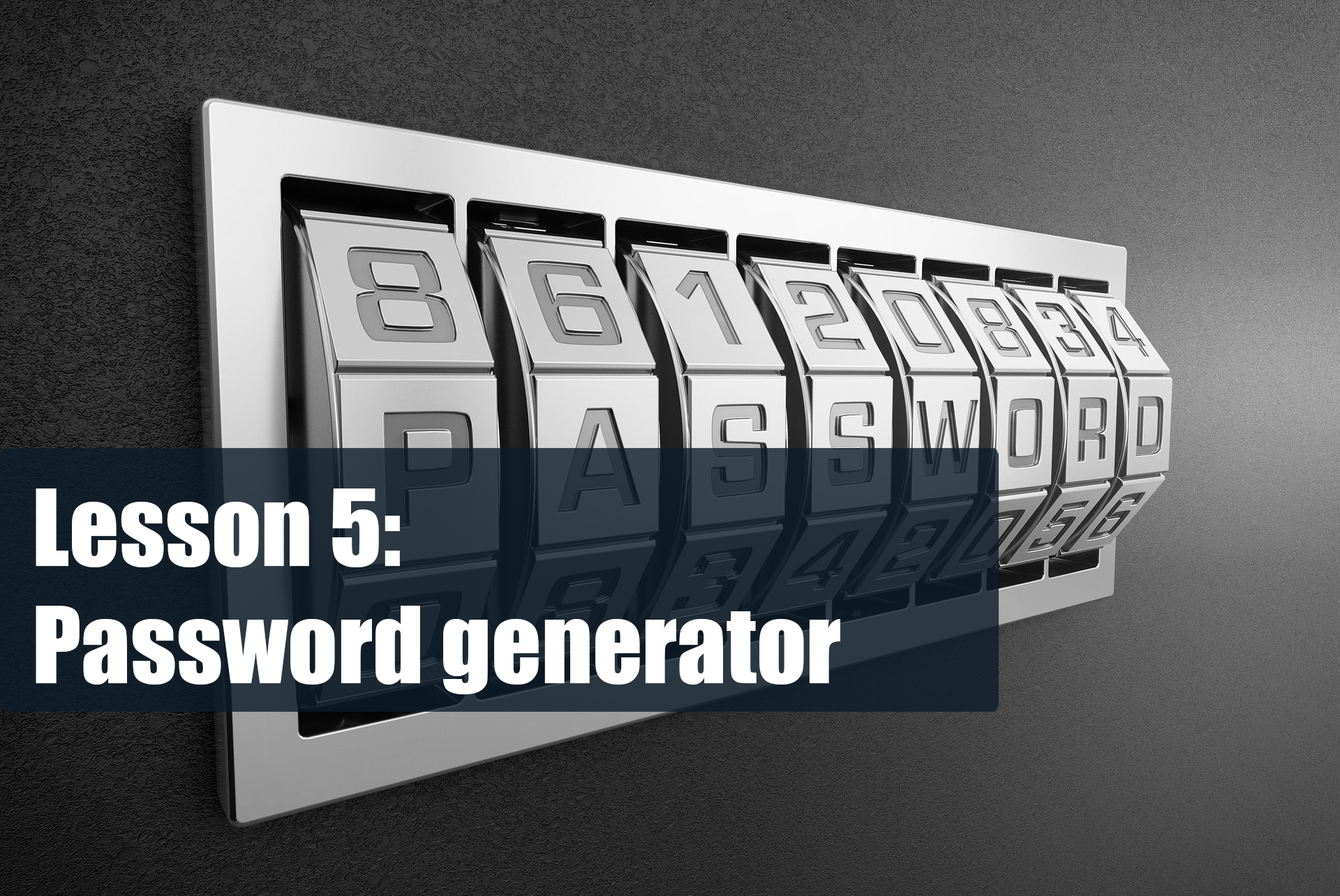
- Set up pseudocode for code design
- Introduce Arrays and Lists
- Design and code a random Password Generator
- OPTIONAL: Create a random fantasy character, game show challenge and shopping list
Videos in this lesson
Arrays
Password generator
Lesson 6: Magic 8 ball
TWO/THREE 45-MINUTE PERIODS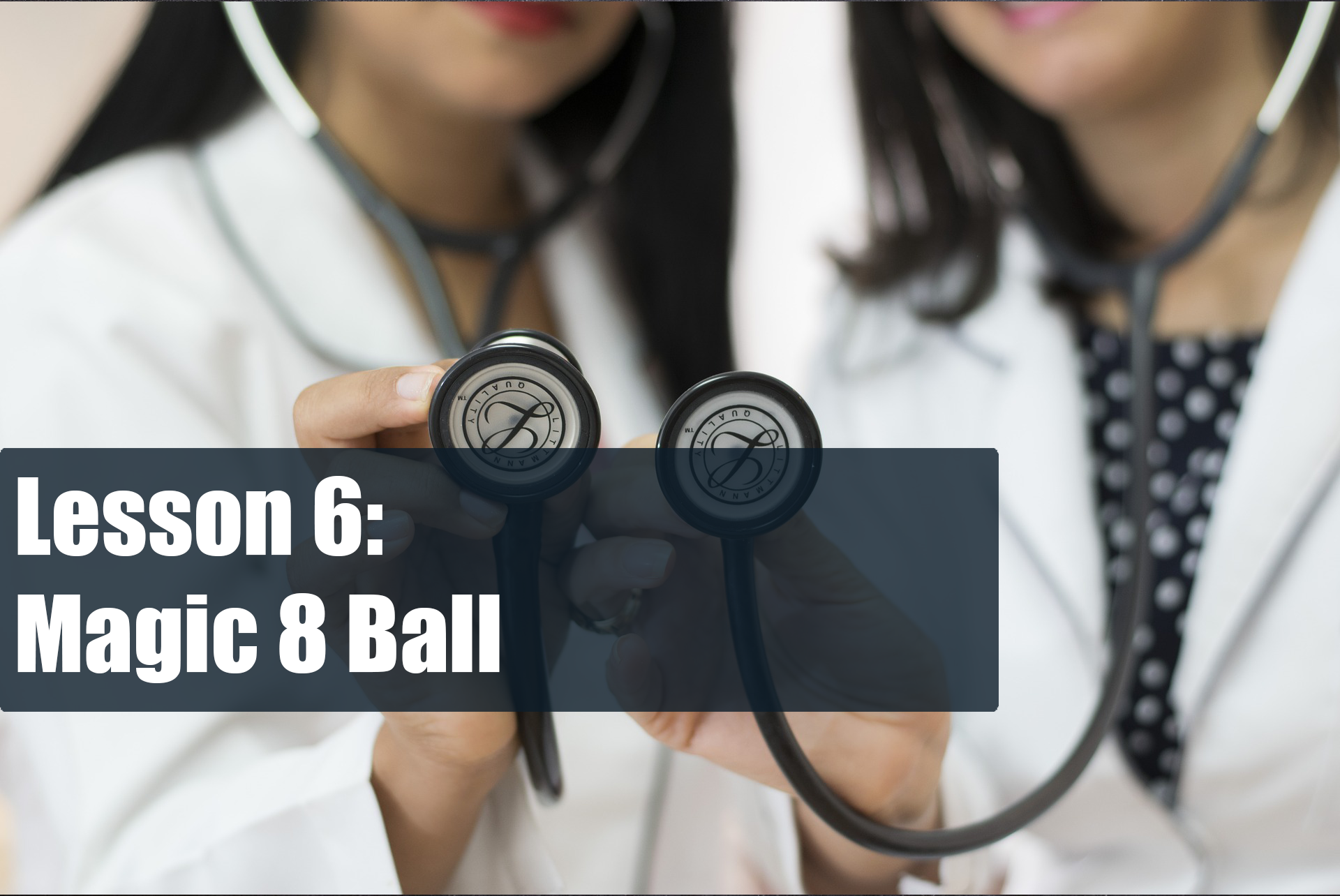
- Introduce the length property for arrays.
- Design and code a Magic 8 Ball to advise you.
Videos in this lesson
Length property
Decision maker and magic 8 ball challenge
Lesson 7: Times tables
TWO/THREE 45-MINUTE PERIODS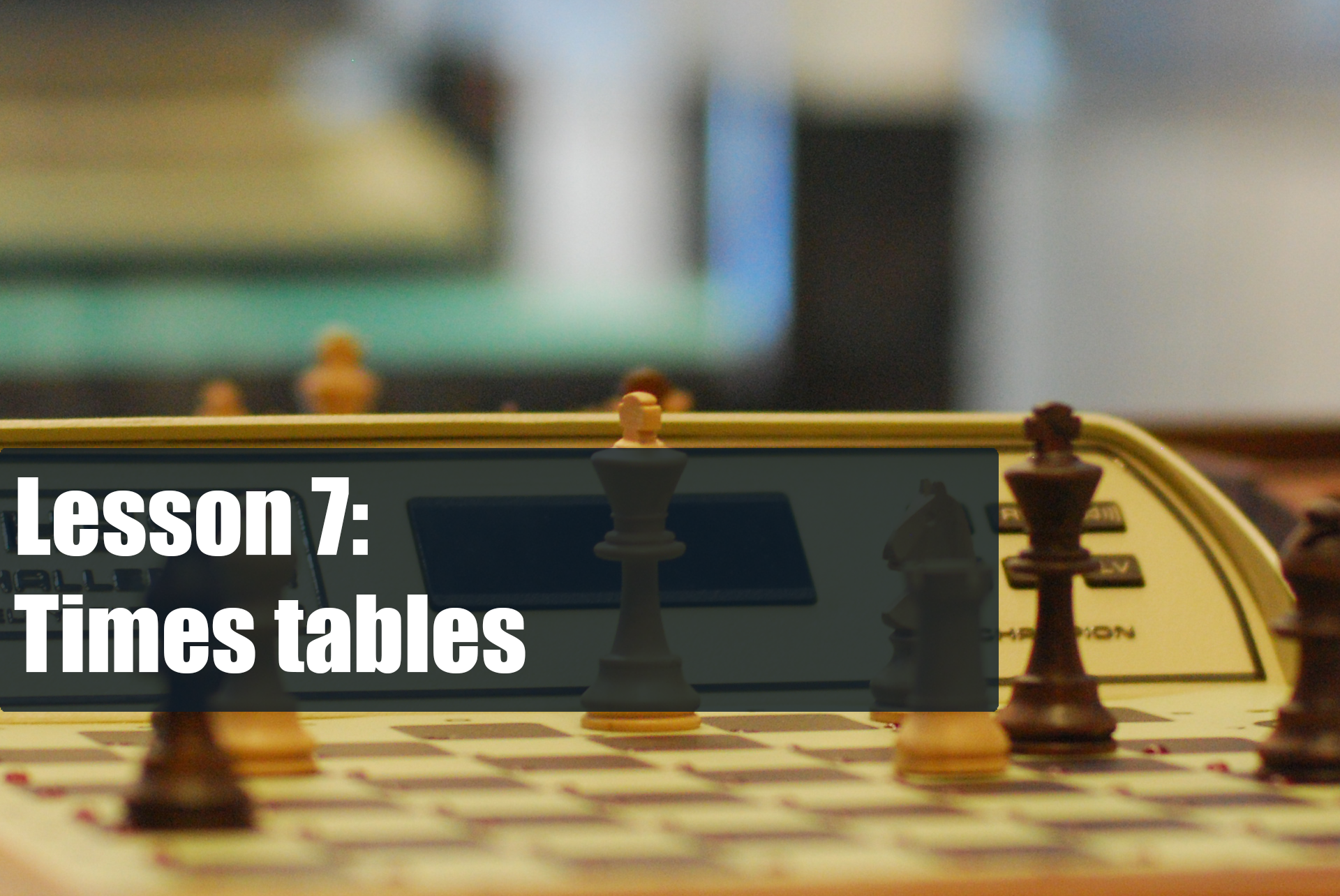
- Introduce iteration (loops).
- Design and code a times table generator for any number.
Videos in this lesson
Loops
Times tables
Lesson 8: Guess the number
TWO/THREE 45-MINUTE PERIODS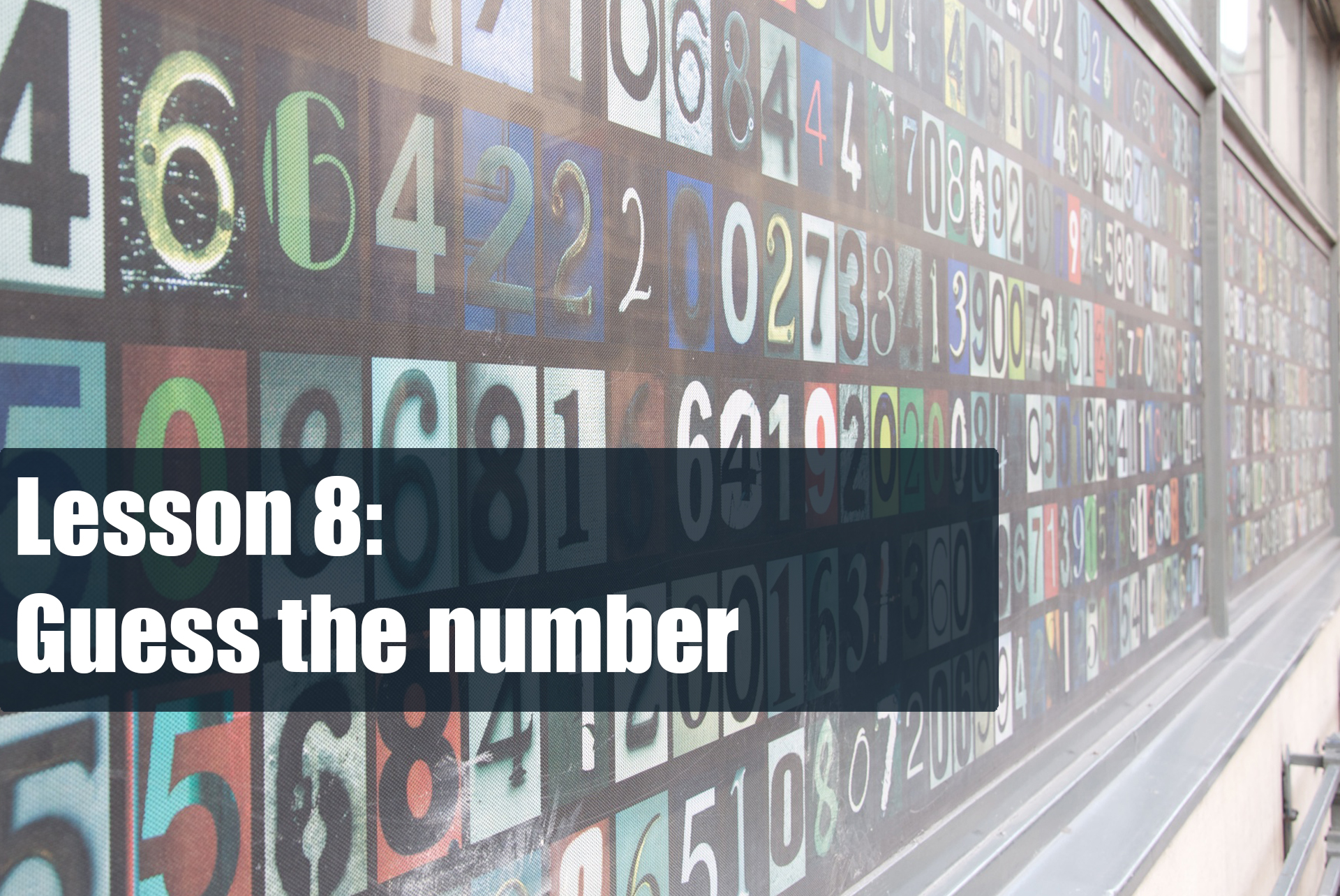
- Learn more about variables and iteration (loops).
- Design and code a higher/lower game, where the player must guess a secret number between 1 and 20.
Videos in this lesson
Guess the number
Vowel replacer
Lesson 9: Loops and arrays combined
TWO/THREE 45-MINUTE PERIODS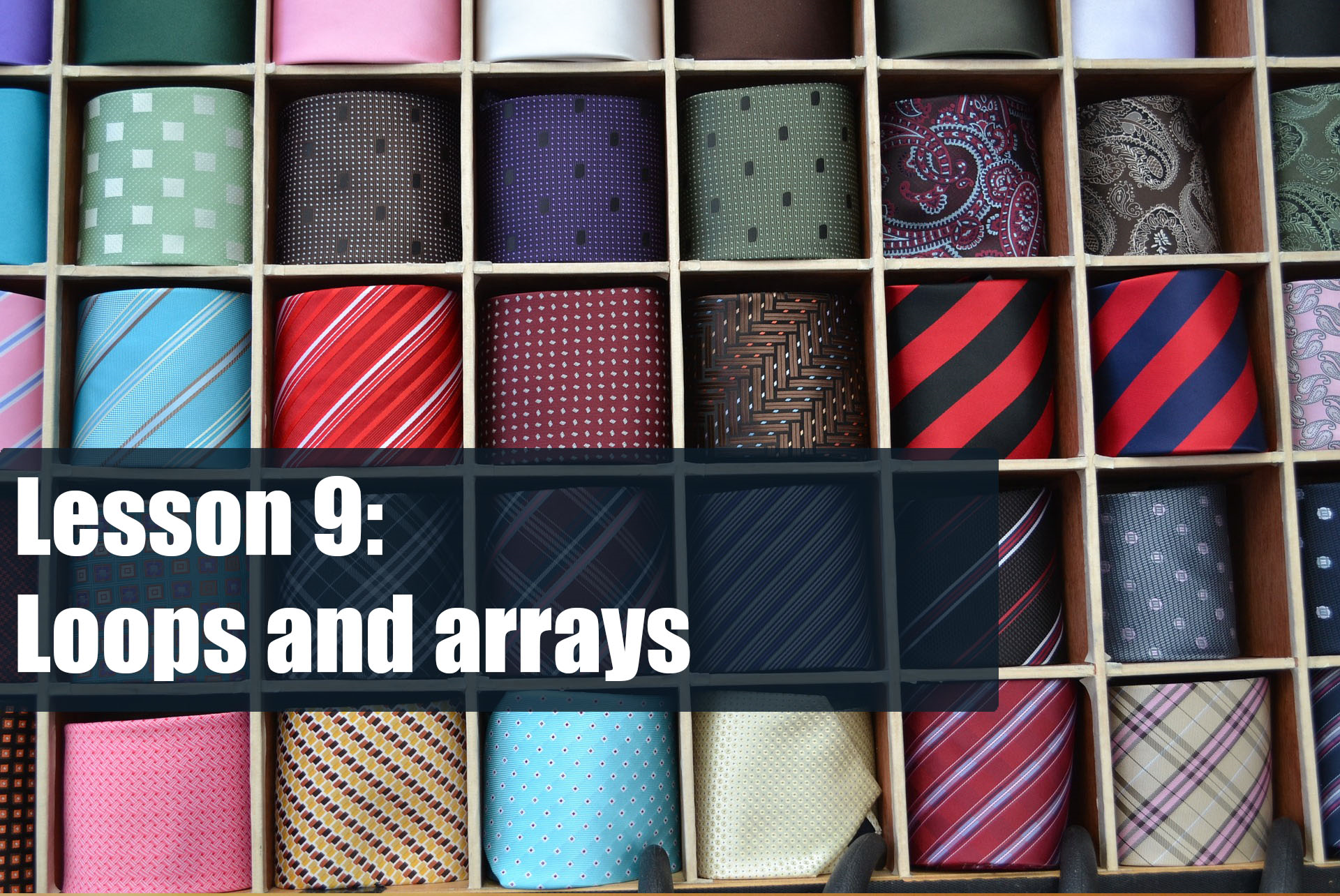
- Discover how loops and arrays work together for powerful programs.
- Build a program to analyse student marks and make useful information.
- OPTIONAL: Challenge yourself to process new data.
Videos in this lesson
Scores
Lesson 10: Simple functions
TWO/THREE 45-MINUTE PERIODS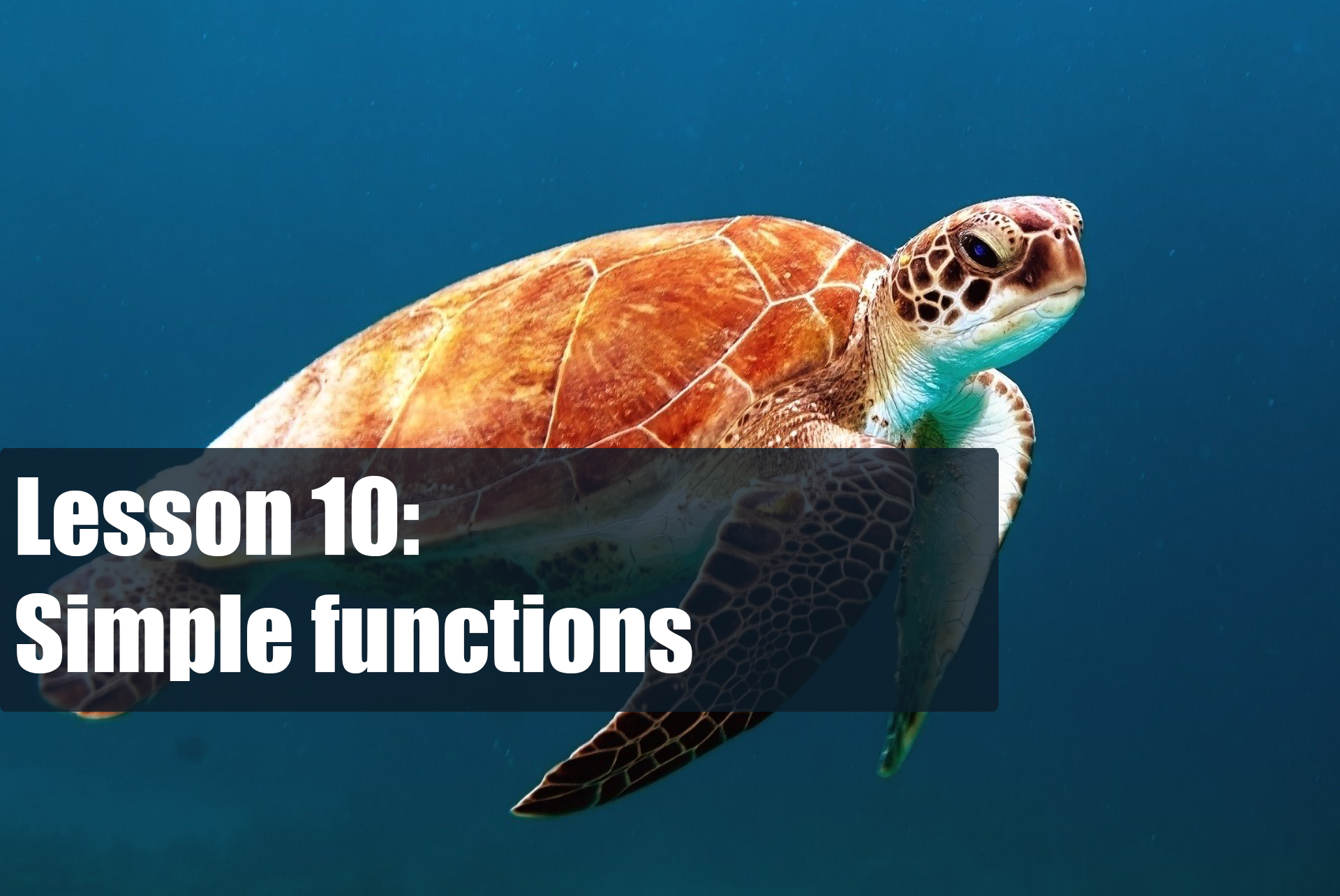
- Set up and practice using turtle graphics in Scratch, Python and JavaScript.
- Create functions without parameters.
- Incorporate loops (iteration) inside a function.
- Discover how functions help organise code.
- OPTIONAL: Challenge yourself to draw a cosmic image
Note: Turtle graphics is an effective context to learn about functions, but examples will also be provided for writing functions outside of the turtle graphics context.
Videos in this lesson
Draw shapes in Scratch
Draw shapes in Python and Javascript
Lesson 11: Adaptable functions with parameters
TWO/THREE 45-MINUTE PERIODS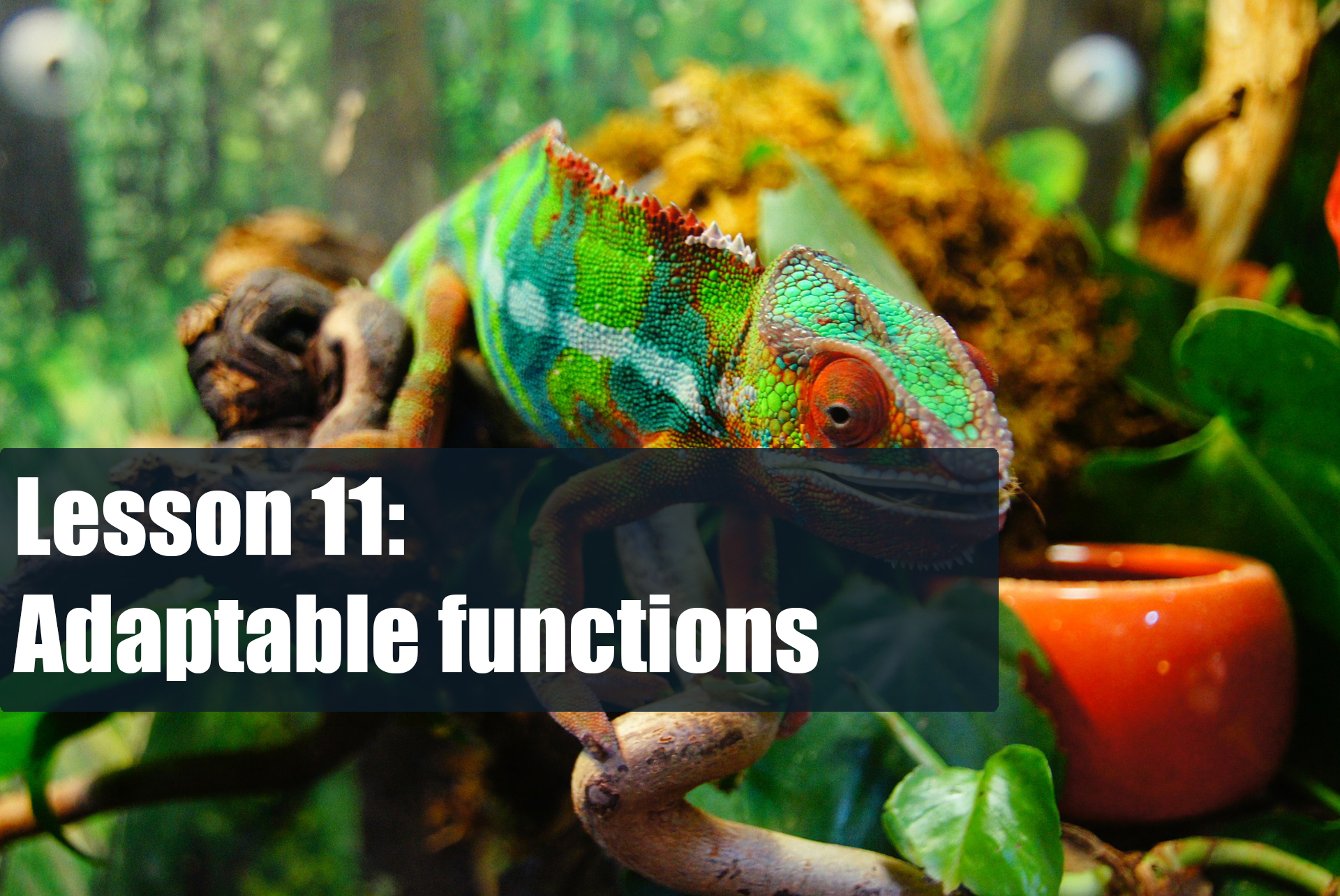
- Create functions that adapt their behaviour thanks to parameters.
- Observe how functions reduce repetition in code.
- OPTIONAL: Challenge yourself to generate a size chart.
Videos in this lesson
Input into functions with parameters and arguments
Reduce repetitive code with functions
Lesson 12: Functions that give back (return values)
TWO/THREE 45-MINUTE PERIODS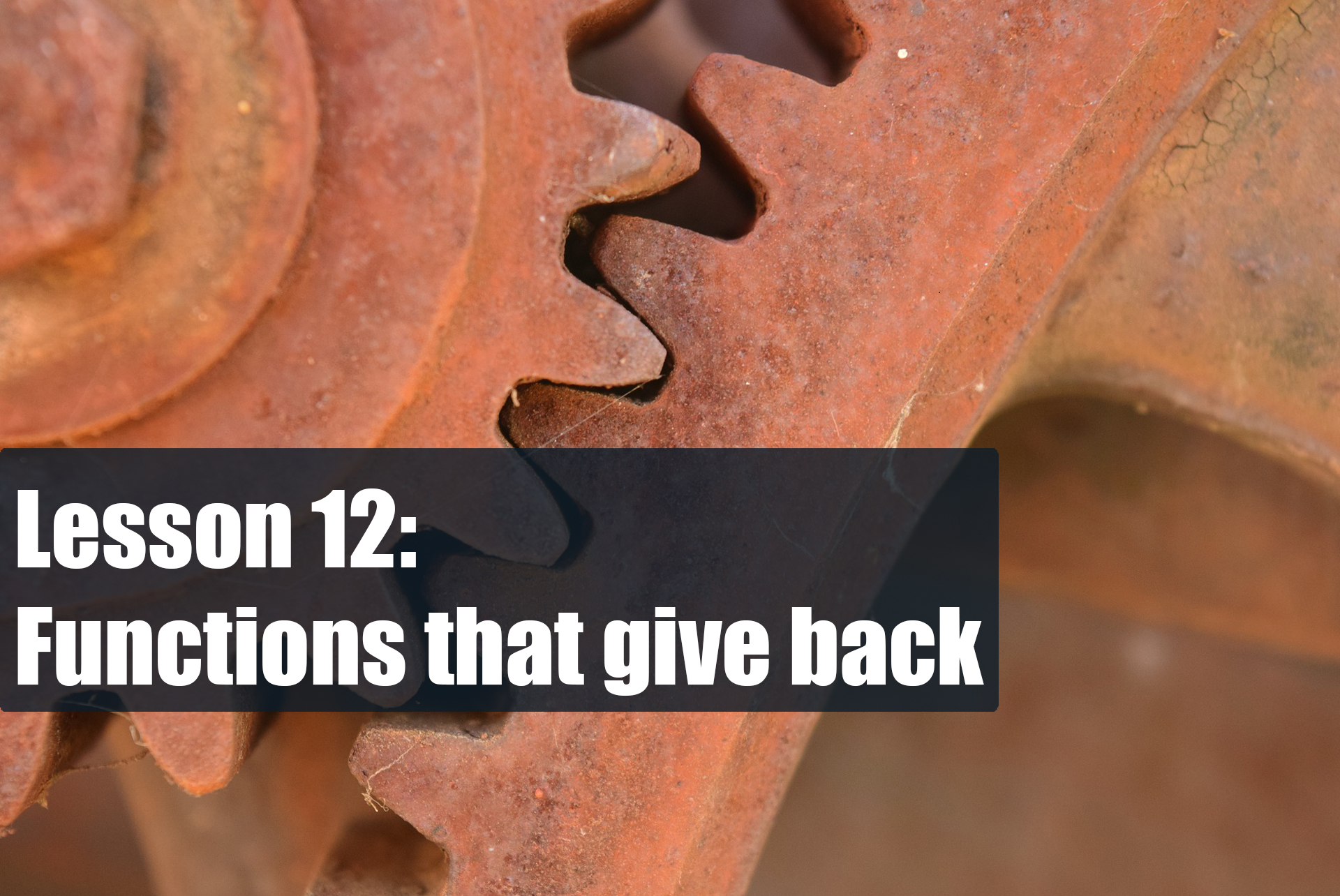
- Identify and describe some of the in-built functions you have been already been using.
- Create functions with return values. They give back an answer, rather than just perform an action.
- Observe how functions are used in Graphical User Interfaces, triggered by user inputs.
- OPTIONAL: Challenge yourself to write a small battle game by building a library of functions.