Off to the movies
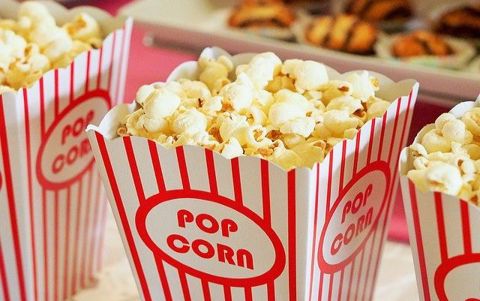
About this lesson
This is a simple Boolean (true/false) application where its asks the user’s age - if you are over 15 then you can watch G and M rated movies - if you are under 15, then you can only watch G rated movies. This lesson was designed in collaboration with Jason Vearing QSITE (Gold Coast Chapter).
Year band: 7-8
Curriculum Links AssessmentCurriculum Links
Links with Digital Technologies Curriculum Area
Strand | Content Description |
---|---|
Processes and Production Skills |
Design the user experience of a digital system (AC9TDI8P07). Design algorithms involving nested control structures and represent them using flowcharts and pseudocode (AC9TDI8P05). Evaluate existing and student solutions against the design criteria, user stories and possible future impact (AC9TDI8P10). |
Learning hook
Imagine an online kiosk that provides a selection of tickets for the movies. The start of the program is to know the user’s age and provide a simple message.
We want to build a simple program that welcomes you to the movie theatre and asks your age. If you enter your age as under 15, then you can only watch G movies. If you enter your age as 15 or over then you can watch G and M rated movies. The program starts by the user clicking a button.
Learning map and outcomes
In this task students will use and develop some important programming skills.
To build the end program a useful approach is to build in stages and encourage students to test as they go. They check for errors as they run the program and trace any errors and modify the code as necessary. Ask them to keep a record of how they have used this approach to build their program.
Before starting to build their program using a text based programming language, ask them to create an algorithm in Pseudocode. This will help them think about the programming requirements and the logical flow.
Learning input
Model and share how to create the Pseudocode with the class. Discuss the types of code they expect to use in their JavaScript program.
PSEUDOCODE (EXAMPLE) START: Select ‘Click here’ button OUTPUT: Welcome to the movies. How old are you? IF INPUT: less than 15 THEN OUTPUT: You can only watch G rated movies. ELSE IF INPUT: 15 or greater THEN OUTPUT: You can watch G and M rated movies. END
Provide students with the link to W3Schools code editor to create and run their JavaScript program. For any students unfamiliar with this code editor demonstrate or ask a student with expertise how to create a simple string, add a button, change text onscreen etc.
Learning construction
Provide a list of steps in building the program to which students can refer, for example:
This can be provided in an online space where students add to this document as required.
Stages | JavaScript (JS) code examples | Comments |
---|---|---|
Create a button |
<button></button> <button>start</button> <button>click here</button> |
// empty button // start button // click here button |
Create an event Turn ‘click here’ button into ‘onclick’ |
<button onclick="myFunction();"> Click here </button> <button onclick="howOld();">Click here</button> |
// general code example // code example with function named as howOld |
Create myfunction and use myfunction |
function howOld() |
// howOld function defined |
Output |
alert(“test”) prompt(“test”) |
// use to test function and show alert box // use to test function and show prompt box |
Declare a variable/Assign a variable |
var answer id=userInput document.getElementbyId |
// variable // define id as user input // look from start of program for id |
String |
“text + var + test” “you are + answer + years of age” |
// string using a variable // string using a variable for user input of age |
Use if/else |
if (answer is <= 15) { document.getElementbyId (‘userInput’).innerHTML = ‘you can only watch G rated movies”; } else { document.getElementbyId (‘userInput’).innerHTML = ‘you can watch G and M rated movies”; } |
// logical condition // executed if condition is true // else is executed if condition is false |
Learning input
For students that require support
Refer to this completed code to assist students that require support to get the code to function as intended.
<h2>Online movie kiosk</h2> <button onclick="howOld();">Click here</button> <p id="userInput">this is where the answer will go</p> <script> function howOld() { var answer = prompt("Welcome to the movie theatre. How old are you?"); if (answer <= 15) { document.getElementById('userInput').innerHTML = "You can only watch G rated movies"; } else { document.getElementById('userInput').innerHTML = "You can watch G and M rated movies"; } } </script>
Compare their code to this code and provide guidance as to what they have entered incorrectly.
To trace errors, check for:
- incorrect use of brackets { and }
- including opening a script and closing the script <script> </script>
- exact use of variable ID in function (sometimes students mistype)
- make sure onclick has an associated function
- include an answer = prompt
- semi colons at end of statements (used to separate statements)
- use of double quotes for all text to show on screen “text”
Challenge
- Continue to build the program and offer a third age option ‘18 and over’.
- Continue to build the program and offer a selection of movies based on the user’s age. The user selects the movie and the correct movie is ‘printed’ onscreen.
- Reuse and modify the code for another purpose. For example, choose a different question which requires two options for an answer, create a function, declare and assign the variables, show output as a string.
Resources
Teacher background
- View this YouTube video tutorial created by Jason Vearing showing how to build the program using Scratch first then showing how to build in stages in JavaScript.
Code editor and programming resources
- Use this website W3Schools My First JavaScript to use the code editor to create and run your program built in JavaScript:
- This JS CheatSheet provides a comprehensive list of example code that can be used to implement a JavaScript program.