Coding a sentimental chatbot in Python
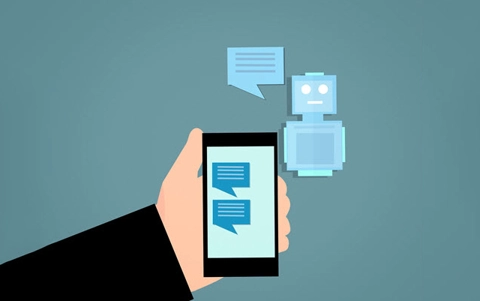
About this course
Incorporating 11 tutorial videos and two informative lecture videos, this learning sequence explores natural language processing, a significant application of artificial intelligence. Teachers and students are led through the coding in Python of a chatbot, a conversational program capable of responding in varied ways to user input, including with the use of smart sentiment analysis.
Year band: 7-8, 9-10
Curriculum Links AssessmentCurriculum Links
Links with Digital Technologies Curriculum Area
Strand | Year | Content Description |
---|---|---|
Processes and Production Skills | 7-8 |
Analyse and visualise data using a range of software, including spreadsheets and databases, to draw conclusions and make predictions by identifying trends (AC9TDI8P02) Define and decompose real-world problems with design criteria and by creating user stories (AC9TDI8P04) Design the user experience of a digital system (AC9TDI8P07) Generate, modify, communicate and evaluate alternative designs (AC9TDI8P08) Design algorithms involving nested control structures and represent them using flowcharts and pseudocode (AC9TDI8P05) Trace algorithms to predict output for a given input and to identify errors (AC9TDI8P06) |
9-10 |
Analyse and visualise data interactively using a range of software, including spreadsheets and databases, to draw conclusions and make predictions by identifying trends and outliers (AC9TDI10P02) Define and decompose real-world problems with design criteria and by interviewing stakeholders to create user stories (AC9TDI10P04) Design and prototype the user experience of a digital system (AC9TDI10P07) Evaluate existing and student solutions against the design criteria, user stories, possible future impact and opportunities for enterprise (AC9TDI10P10) Design algorithms involving logical operators and represent them as flowcharts and pseudocode (AC9TDI10P05) Implement, modify and debug modular programs, applying selected algorithms and data structures, including in an object-oriented programming language (AC9TDI10P09) |
Assessment
Many of the tutorial videos provide tinker tasks, where students are challenged to modify the demonstrated code for a new purpose. Short, fresh coding challenges are also presented after each tutorial.
Finally, two major challenges are presented at the end, using the skills covered throughout this learning sequence. The first challenge has the code design completed, while the other requires students to create a program design before implementing a coded program.
In assessing code in languages like Python, consider a rubric that brings in important skills for general purpose programming.
Course structure
Introduction to chatbots
View the video Lecture – Intro to chatbots to discover the range of applications of chatbots incorporating natural language processing in the world today.
Lectures are primarily intended for a teacher audience, but you may choose to re-watch the video with students.
Tutorial 1: Nicknames
View the video Tutorial – Nicknames. In this tutorial, you will code a program to make the computer give you a nickname.
(Completed code up to this point.)
Tinker challenges
Click here for the tinker challenges presented in the video together with their solutions.
Fresh code challenge
Now that you can write a program to give someone a nickname, write a program to make someone’s name sound like an Ancient Roman name, as in the running example below.
Harry
If you were an Ancient Roman, your name might be Harryus Maximus IV.
Click here for a solution to the fresh code challenge.
Tutorial 2: Simple conditional response
View the video Tutorial – ifelse. In this tutorial, you will code a program that asks a question, reads the response and decides how to answer back.
(Completed code up to this point.)
Tinker challenges
Click here for the tinker challenges presented in the video together with their solutions.
Fresh code challenge
Now that you can use if-else, write a short quiz as in the running example below.
red
Correct!
What is the capital of Uzbekistan?
tashkent
Incorrect. It should be spelled with a capital letter.
What word describes a government by the wealthy?
plutocracy
Correct!
Remember:
- You should tell the user if they got a question right or wrong.
- The capital city must be spelled with a capital first letter, but the other questions should not be case sensitive.
Click here for a solution to the fresh code challenge.
Tutorial 3: Looking for keywords
View the video Tutorial – membership. In this tutorial, you will code a program to make the chatbot look for keywords in the user’s responses.
(Completed code up to this point.)
Tinker challenges
Click here for the tinker challenges presented in the video together with their solutions.
Fresh code challenge
Now that you can find words in a user response, you can also find letters. Write a challenge for the user as in the two running examples below.
The quick brown fox jumped over the lazy dog.
Too bad! Your sentence has an e in it.
Try writing a sentence without using the letter e.
How much wood would a woodchuck chuck if a woodchuck could chuck wood?
You did it!
Click here for a solution to the fresh code challenge.
Tutorial 4: Natural ways to ask a question
View the video Tutorial – random-questions. In this tutorial, you will give your chatbot more ways to ask questions, so that it seems more natural.
(Completed code up to this point.)
Tinker challenges
Click here for the tinker challenges presented in the video together with their solutions.
Fresh code challenge
Now that you can choose a random element from a list, write a program to allow the chatbot to tell you about its day. The chatbot will randomly choose one of five things to say, as in the running examples below.
How are you going?
Great! I’m crushing it!
Hey, now it is your turn to ask me how my day is going.
How are you going?
Terrible. First I spilled my breakfast this morning, and my bus left early.
Click here for a solution to the fresh code challenge.
Tutorial 5: A list of positive responses
View the video Tutorial – random-questions. In this tutorial, you will give your chatbot more ways to ask questions, so that it seems more natural.
(Completed code up to this point.)
Tinker challenges
Click here for the tinker challenges presented in the video together with their solutions.
Fresh code challenge
Tutorial 5 introduced Boolean, a special data type that can only be one of two things: true or false. Boolean variables are often used as flags to remember whether something has happened or not.
Use the pseudocode below to write a program that uses a Boolean named success to keep a user guessing until they can supply a suitable answer.
countries ← ['Argentina', 'Australia', 'Chile', 'France', 'New Zealand', 'Norway', ‘United Kingdom']
Say 'To end this program, you must name a country that has an original territorial claim in Antarctica. Type as much as you like.'
success ← False
While success = False
Say 'Have a try.'
response ← input from user
For each country in countries
If response contains country
success ← True
End If
End For
End While
Say 'Well done! You made it to the end of this program!'
END
Click here for a solution to the fresh code challenge.
Lecture: Sentiment analysis
View the video Lecture – sentimentAnalysisLecture to:
- discover interesting connections between linguistics and digital technologies
- import a Python module that makes it easy to incorporate sentiment analysis into your own programs
- explore two key aspects of text sentiment: polarity and subjectivity.
Lectures are primarily intended for a teacher audience, but you may choose to re-watch the video with students.
Tutorial 6: Respond to the user's sentiment
View the video Tutorial – sentiment. In this tutorial, you will experiment with the TextBlob library to respond to the sentiment in a user’s response.
(Completed code up to this point.)
Tinker challenges
Click here for the tinker challenges presented in the video together with their solutions.
Fresh code challenge
Now that you have seen TextBlob in action, write a program to judge the user on how subjective their responses are. The program should be pretty harsh! It should complain about even a small amount of subjectivity. See the running examples below.
I really like soccer.
If you want to be like a computer, you must be less subjective.
Hi. Try to say something without much subjectivity.
The weather is clement today.
That’s pretty good. Well done!
Click here for a solution to the fresh code challenge.
Major project
View the video Overview of project to learn how we will bring together the pieces from the tutorials so far.
(Completed code up to this point.)
In this section, we make introductory conversation about the user’s name.
In this section, we add a friendly enquiry and respond to the user’s sentiment.
In this section, we choose a random question from a list of choices.
In this section, we respond to the user’s answer using sentiment analysis.
In this section, we generate a friendly goodbye. Extra improvements are made to the overall program.
Final design and coding challenges
These challenges potentially could be used for optional assessment. They use the same code structures and statements covered in this learning sequence, including the TextBlob module.
Challenge 1: Automated phone machine
This challenge requires code implementation only. Design is supplied in the form of running examples and pseudocode. Click here for a solution to the challenge.
Betty’s Blinds is a popular seller of blinds, curtains and carpets. With so many customers, the managers have decided to invest in an automated phone machine that will direct callers to the correct department.
The system will listen for keywords that the customer says, then direct the call to the Blinds Department, Curtains Department or Carpets Department. Also, it will attempt to detect if a customer is irate and immediately direct the call to a manager.
Running examples.
Welcome to Betty's Blinds. So we can direct your call, please say what your call is about.I need new curtains.
Directing you to our Curtains Department now.
Welcome to Betty's Blinds. So we can direct your call, please say what your call is about.
Do you sell Venetians?
Directing you to our Blinds Department now.
Welcome to Betty's Blinds. So we can direct your call, please say what your call is about.
I'm very angry. Your curtains are rubbish.
Directing you to one of our managers now.
Welcome to Betty's Blinds. So we can direct your call, please say what your call is about.
Do you sell car insurance here?
Sorry, I couldn't determine what your call is about. Directing you to one of our managers now.
Pseudocode.
blinds_words ← ['blinds', 'venetian', 'venetians', 'roman', 'vertical', 'roller', 'aluminium']
curtains_words ← ['curtain', 'curtains', 'pleated', 'eyelet', 'eyelets', 'rod', 'rods']
carpets_words ← ['carpet', 'carpets', 'rug', 'rugs', 'underlay', 'floor', 'floors']
// Give welcome.
Say 'Welcome to Betty's Blinds. So we can direct your call, please say what your call is about.'
response ← input from user
// Use a variable to keep where to direct the call.
call_destination ← 'unknown'
// First check sentiment in case of irate customer.
If polarity of response < -0.2
call_destination ← 'manager'
// Search the response for a word relating to blinds.
If call_destination = 'unknown'
For each word in blinds_words
If response contains word
call_destination ← 'blinds'
// Search the response for a word relating to curtains.
If call_destination = 'unknown'
For each word in blinds_words
If response contains word
call_destination ← 'curtains'
# Search the response for a word relating to blinds.
if call_destination = 'unknown'
For each word in blinds_words
If response contains word
call_destination ← 'carpets'
# Finally, direct the call.
if call_destination = 'blinds'
Say 'Directing you to our Blinds Department now.'
Else If call_destination = 'curtains'
Say 'Directing you to our Curtains Department now.'
Else If call_destination = 'carpets'
Say 'Directing you to our Carpets Department now.'
Else If call_destination = 'manager'
Say 'Directing you to one of our managers now.'
Else
Say ‘Sorry, I couldn't determine what your call is about. Directing you to one of our managers.’
Challenge 2: Restaurant review analyser
This challenge requires code design and implementation. Along with early experimentation with TextBlob, students should produce a flowchart and/or pseudocode before completing their solution. Click here for a solution to the challenge.
Restaurants receive thousands of comments from happy and unhappy customers on their websites and through social media. These are usually one paragraph, and often one sentence only.
The challenge is to turn this qualitative data (typed reviews) into something quantitative, a numerical figure like a star rating from 1 to 5. This can be done manually, but new natural language processing might help us to automate the process.
Your software will:
- allow a user to type or paste a comment
- use natural language processing to perform sentiment analysis on the comment
- attempt to produce a star rating from 1 to 5 to reflect that comment, based on how positive or negative it is.
Example review comments are given below. You may have to experiment a little to find the most reliable way to produce your star rating.
- The food was average.
- Terrible restaurant.
- The lasagne was OK.
- The beef consommé was pretty bad.
- Fabulous. Everything was perfect.
Extensions
- Could you improve the program to accept multiple reviews and calculate an average star rating for the restaurant?
Resources
- Python cheat sheet (from Grok Learning)
- Visual to text coding series of lessons with videos and exercises to help you and your class transition from visual coding (eg Scratch) to general purpose programming (eg Python and JavaScript)
- Natural language processing on Wikipedia